Top 10 JavaScript Challenges for Advanced Coders | Expert Tips & Solutions
10 JavaScript Challenges for Advanced Coders
JavaScript remains an integral part of web development, and mastering its complexities elevates a coder’s prowess. As a coder progresses, tackling intricate algorithms becomes pivotal for honing skills and problem-solving abilities. Let’s delve into ten challenging JavaScript algorithms tailored for advanced coders, offering insights, solutions, and a thorough understanding of their implementations.
1. Reversing a String in JavaScript
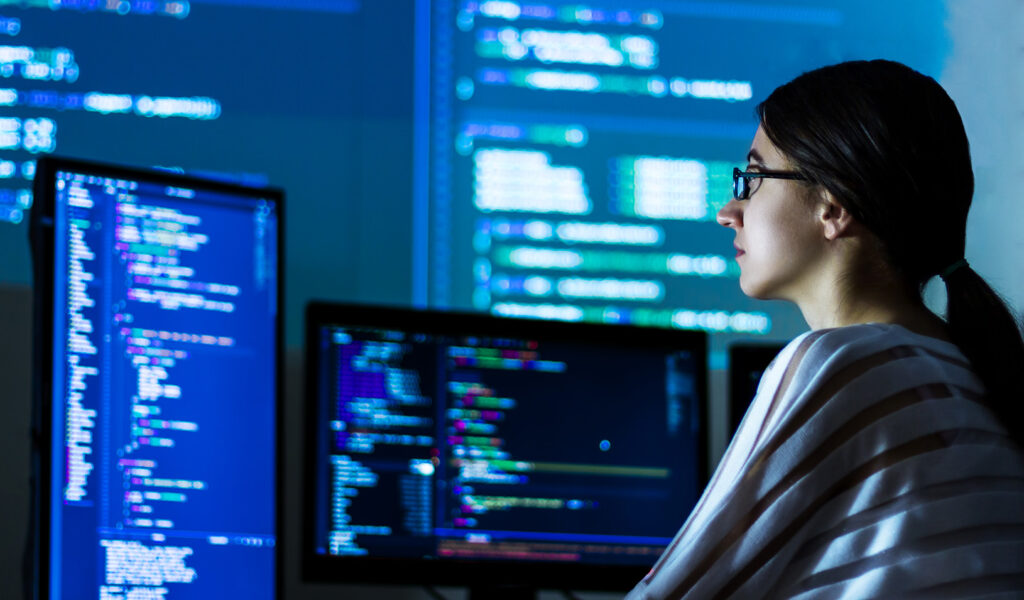
Unraveling the Challenge
Reversing a string is a fundamental task that often conceals intricate solutions. Using recursion to reverse strings or employing built-in methods like ‘split()‘, ‘reverse()‘, and ‘join()‘ can unravel this puzzle.
Expert Solution
Consider this JavaScript snippet using a recursive approach:
function reverseString(str) { if (str === '') { return ''; } else { return reverseString(str.substr(1)) + str.charAt(0); } }
2. Implementing a Binary Search Algorithm
Navigating the Complexity
Binary search is a pivotal algorithm in computer science, optimizing search processes. For advanced coders, grasping its nuances through iteration or recursion is crucial.
Advanced Strategy
Here’s an iterative approach in JavaScript:
function binarySearch(arr, target) { let left = 0; let right = arr.length - 1; while (left <= right) { let mid = Math.floor((left + right) / 2); if (arr[mid] === target) { return mid; } else if (arr[mid] < target) { left = mid + 1; } else { right = mid - 1; } } return -1; }
3. Understanding Memorization in Dynamic Programming
Tackling Dynamic Challenges
Memorization, a concept integral to dynamic programming, optimizes recursive algorithms by storing computed values. Implementing memorization in JavaScript can significantly enhance algorithm efficiency.
Implementation Guide
Utilize memorization in a recursive Fibonacci sequence:
let memo = {}; function fibonacci(n) { if (n in memo) { return memo[n]; } if (n <= 2) { return 1; } memo[n] = fibonacci(n - 1) + fibonacci(n - 2); return memo[n]; }
4. Solving the Tower of Hanoi Puzzle
Conquering Puzzle Complexity
The Tower of Hanoi problem involves moving disks from one rod to another while adhering to specific constraints. Implementing a recursive solution in JavaScript can conquer this challenge.
Expert Approach
Let’s illustrate a JavaScript solution:
function towerOfHanoi(n, source, auxiliary, destination) { if (n === 1) { console.log(`Move disk 1 from rod ${source} to rod ${destination}`); return; } towerOfHanoi(n - 1, source, destination, auxiliary); console.log(`Move disk ${n} from rod ${source} to rod ${destination}`); towerOfHanoi(n - 1, auxiliary, source, destination); }
5. Solving the Knapsack Problem
Complex Bagging Conundrum
The Knapsack problem involves maximizing value while considering item weights and a limited capacity. Dynamic programming is pivotal in achieving an optimal solution.
Advanced Methodology
Here’s a JavaScript implementation:
function knapsack(weights, values, capacity, n) { if (n === 0 || capacity === 0) { return 0; } if (weights[n - 1] > capacity) { return knapsack(weights, values, capacity, n - 1); } else { return Math.max( values[n - 1] + knapsack(weights, values, capacity - weights[n - 1], n - 1), knapsack(weights, values, capacity, n - 1) ); } }
6. Implementing Depth-First Search (DFS)
Navigating Graph Traversal
DFS is a crucial algorithm for graph traversal. Understanding its mechanics and implementing recursive or iterative approaches is pivotal.
Implementation Insight
Implementing DFS in JavaScript for graph traversal:
class Graph { constructor() { this.adjList = new Map(); } addVertex(v) { this.adjList.set(v, []); } addEdge(v, w) { this.adjList.get(v).push(w); } // DFS traversal dfs(startingNode) { let visited = {}; this._dfsHelper(startingNode, visited); } _dfsHelper(vertex, visited) { visited[vertex] = true; console.log(vertex); let neighbors = this.adjList.get(vertex); for (let neighbor of neighbors) { if (!visited[neighbor]) { this._dfsHelper(neighbor, visited); } } } }
7. Solving the Travelling Salesman Problem
Conquering Optimization Challenges
The Travelling Salesman Problem involves finding the shortest route visiting all cities exactly once and returning to the original city. Applying dynamic programming is key to finding optimal solutions.
Advanced Strategy
A JavaScript implementation using dynamic programming:
function travellingSalesman(graph, start) { let nodes = Object.keys(graph); let visited = []; visited[start] = true; let result = [start]; let minDist = Infinity; let next = null; for (let i = 0; i < nodes.length; i++) { if (!visited[i]) { let current = start; let dist = 0; let temp = [start]; while (temp.length < nodes.length) { let k = -1; let min = Infinity; for (let j = 0; j < nodes.length; j++) { if (!visited[j] && graph[current][j] < min) { min = graph[current][j]; k = j; } } if (k === -1) { break; } temp.push(k); visited[k] = true; dist += graph[current][k]; current = k; } if (temp.length === nodes.length && graph[current][start] < Infinity) { dist += graph[current][start]; temp.push(start); if (dist < minDist) { minDist = dist; result = temp; } } } visited = []; visited[start] = true; } return result; }
8. Understanding Dynamic Programming: Longest Common Subsequence
Deciphering Sequence Complexity
The Longest Common Subsequence problem involves finding the longest subsequence present in given sequences. Implementing a dynamic programming solution is pivotal for efficiency.
Advanced Approach
Here’s a JavaScript implementation of the LCS problem:
function longestCommonSubsequence(text1, text2) { const m = text1.length; const n = text2.length; const dp = new Array(m + 1).fill(0).map(() => new Array(n + 1).fill(0)); for (let i = 1; i <= m; i++) { for (let j = 1; j <= n; j++) { if (text1[i - 1] === text2[j - 1]) { dp[i][j] = dp[i - 1][j - 1] + 1; } else { dp[i][j] = Math.max(dp[i - 1][j], dp[i][j - 1]); } } } let i = m; let j = n; let result = ''; while (i > 0 && j > 0) { if (text1[i - 1] === text2[j - 1]) { result = text1[i - 1] + result; i--; j--; } else if (dp[i - 1][j] > dp[i][j - 1]) { i--; } else { j--; } } return result; }
9. Solving the Coin Change Problem
Navigating Coin Selection Challenges
The Coin Change problem involves determining the number of ways to make change for a particular amount using a given set of coins. Utilizing dynamic programming is fundamental for comprehensive solutions.
Expert Methodology
Implementing a JavaScript solution:
function coinChange(coins, amount) { const dp = new Array(amount + 1).fill(Infinity); dp[0] = 0; for (let i = 1; i <= amount; i++) { for (let j = 0; j < coins.length; j++) { if (coins[j] <= i) { dp[i] = Math.min(dp[i], dp[i - coins[j]] + 1); } } } return dp[amount] === Infinity ? -1 : dp[amount]; }
10. Implementing the Sieve of Eratosthenes
Mastering Prime Number Generation
The Sieve of Eratosthenes is a classic algorithm for generating all prime numbers smaller than a given number. Applying this algorithm in JavaScript showcases its efficiency.
Implementation Insight
A JavaScript implementation for finding prime numbers:
function sieveOfEratosthenes(n) { let primes = []; let isPrime = new Array(n + 1).fill(true); isPrime[0] = isPrime[1] = false; for (let i = 2; i * i <= n; i++) { if (isPrime[i]) { for (let j = i * i; j <= n; j += i) { isPrime[j] = false; } } } for (let i = 2; i <= n; i++) { if (isPrime[i]) { primes.push(i); } } return primes; }
Conclusion
Mastering complex algorithms in JavaScript is an exhilarating journey for advanced coders. These ten challenges encapsulate a spectrum of problem-solving, enhancing expertise while unraveling the intricacies of JavaScript algorithms. Pushing boundaries and exploring various strategies fortifies coding capabilities, enabling coders to craft elegant, efficient solutions. Marketing Edge provide JavaScript’s services for web development.
FAQs
Q1: Are these challenges suitable for intermediate-level coders?
A1: These challenges are tailored for advanced coders aiming to fortify their skills in JavaScript algorithms. For intermediate-level coders, tackling simpler variations before diving into these challenges is advisable.
Q2: How can I assess my solutions to these challenges?
A2: Testing your solutions using different test cases, benchmarking performance, and comparing against optimized approaches aids in assessing and refining your solutions.
Q3: Are there resources available for further exploration of these algorithms?
A3: Yes, various online platforms, coding communities, and books offer in-depth explanations, practice problems, and discussions on these algorithms, fostering continuous learning and exploration.
Ali Zahid
Awesome! Very helpful content for advanced developers.
Thanks for providing such amazing information about JavaScript..
Ahmed Hassan
Thank you Ali Zahid